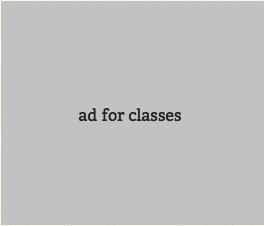
ActiveAdmin with Existing Devise Authentication
I’m modifying an existing Rails app to use ActiveAdmin — one of the new generation of Rails admin consoles (aka dynamic scaffolding for your models). I like its approach to customization, where it assumes we’ll need to. The docs are well-written and clear, but fairly thin; however, I found a number of good resources published elsewhere:
- RailsCast
- Net Tuts+ tutorial
- Setting Up Active Admin on Heroku with Rails 3.1 and Cedar
- Sample Admin Page Gist
With a little help from stackoverflow, I got it working with my existing user model.
Note: ActiveAdmin uses kaminari, instead of will_paginate. There’s some discussion of making it more compatible, but I just switched to kaminari.
add to Gemfile:
1 |
gem 'activeadmin' |
Then on the command line:
1 2 |
bundle install
rails generate active_admin:install --skip-users |
in your config/initializers/active_admin.rb you configure your devise routes, instead of these:
1 2 3 |
config.current_user_method = :current_admin_user
config.logout_link_path = :destroy_admin_user_session_path
config.logout_link_method = :get |
if you are using the default name “user” for existing Devise authentication, change to:
1 2 3 |
config.current_user_method = :current_user
config.logout_link_path = :destroy_user_session_path
config.logout_link_method = :delete |
Then define:
1 2 3 |
def authenticate_admin_user!
redirect_to new_user_session_path unless current_user.try(:is_admin?)
end |
You could put it into the initializer, but I put this in my ApplicationController which seemed a somewhat better place for it.
I use cucumber to test that I’ve got everything configured correctly:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
Scenario: As a regular user who is signed in, I am redirected to the homepage
Given I am signed in
When I go to /admin
Then I should be on /
Scenario: If I am not signed in, I am redirected to login
When I go to /admin
Then I should be on /users/sign_in
Scenario: As an admin user, I can see the admin dashboard
Given I am signed in as an admin
When I go to /admin
Then I should be on /admin
And I should see "Dashboard" |
Steps are defined like this
1 2 3 4 5 6 7 8 9 |
When /^I am signed in$/ do
user = Factory(:user)
login_as user
end
When /^I am signed in as an admin$/ do
user = Factory(:user, :admin => true)
login_as user
end |
Using Warden test helpers, which I set up in features/support/env.rb
1 2 3 4 5 6 7 |
include Warden::Test::Helpers
AfterConfiguration do |config|
:
Warden.test_reset!
Warden.test_mode!
end |
One Comment
I did all the steps but now all the logins fail with a 401 error message.